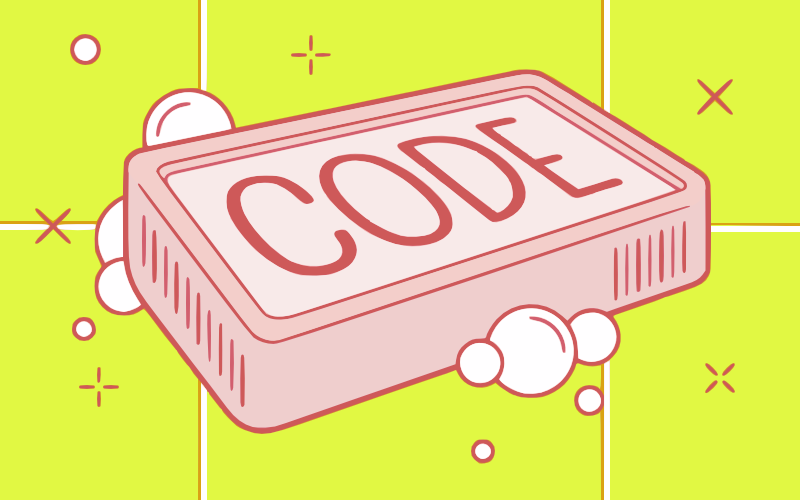
1.有意義的命名
變數與函式命名
1 | // Don't ❌ |
有意義且駝峰大小寫
布林變數
1 | // Don't ❌ |
會加入形容動作
重複的詞彙
1 | // Don't ❌ |
有意義的常數
1 | // Don't ❌ |
全域性常量可以採用SCREAMING_SNAKE_CASE
風格命名。
2. 簡單函式
預設值當參數
1 | // Don't ❌ |
避免重複的事
1 | // Don't ❌ |
可以看起來有相似的功能就可以併起來,避免重複做一樣的事情
一次一件事
1 | // Don't ❌ |
在同個函式裡做太多不同的事,會導致可讀性變差。
避免過多的參數
1 | // Don't ❌ |
某些情況下可直接引入物件並直接解構賦值
,可避免過多的參數
避免副作用
1 | // Don't ❌ |
大多數情況下都應該保持純函式。副作用可能會修改共享狀態和資源,導致一些奇怪的問題。
避免使用布林標誌作為參數
1 | // Don't ❌ |
函式含有布林值的參數意味這個函式是可以被簡化的。
Promise aysnc awit 處理非同步
1 | // Don't ❌ |
callback hell
是很可怕的
3. 條件語句
使用非負條件
1 | // Don't ❌ |
某些判斷可簡寫
1 | // Don't |
if else避免過多分支
1 | // Don't |
儘早return
會使你的程式碼線性化、更具可讀性且不那麼複雜。
優先使用 map 而不是 switch 語句
1 | // Don't ❌ |
減少複雜度且提升效能。
只有if的話
1 | // Don't ❌ |
善用三元表達式
1 | // Don't ❌ |
1 | function example(…) { |
如果有很多else if,可考慮改成三元表達式
三元好棒,但不要走火入魔
1 | // Don't ❌ |
有時不是寫得越短越好,有些比較複雜的邏輯不用硬要追求很短,
一般來說寫的越好的Code,通常越好維護
4. 其他
解構賦值
1 |
|
把0縮寫
1 | // Don't ❌ |
避免過多的註解
1 | // Don't ❌ |
有人說最乾淨的code就是不下註解
。
遵守有意義的命名
,只註解複雜的程式邏輯。
小結
不是說一定怎麼寫會比較好,但前人的經驗告訴我們,在一般的情況下遵從以上的原則,可以提升程式碼的可讀性,更好維護。
參考資料
如何寫出乾淨的 JavaScript 程式碼
5 Best Practices for Writing Clean JavaScript
10 Clean code examples (Javascript).